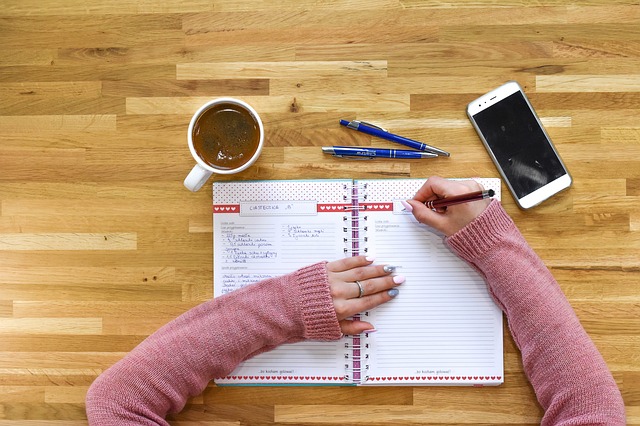
Note-Taking with Vim
By: Roger Creasy
I am experimenting with note-taking/journaling using Vim. I use Vim for almost all other text-based activities, so, why not? I have used Evernote for many years, and have a ton of content there. But, I am looking for more security and control.
I added a function to my .aliases file, which is sourced by my .zshrc file (the function should work fine for Bash as well). This function can do several things:
- Create a file named with the current date, write a date stamp to the top of the file, then open it in Vim.
- Create a file with a name of your choosing, then open it in Vim
- Search your note directory for all note files, and generate a preview of the currently selected (not opened) file
- Search all of your notes for a text string
Here is the code, followed by a line-by-line explanation:
cd /home/roger/Documents/notes
if [ $# -gt 0 ]; then
case $1 in
-n)
case "$2" in
"")
NAME=$(date +"%F")
TODAY=$(date +"%A-%b-%d-%Y")
touch $NAME
echo "$TODAY \n\n" >> $NAME
vim $NAME
;;
*)
NAME=$2
touch $NAME
vim $NAME
;;
esac;;
-s)
case "$2" in
"")
vim `fzf --preview="cat {}" --preview-window=right:70%:wrap | sort -k9`
;;
*)
vim `grep -rnw '/home/roger/Documents/notes' -e $2 | cut -f1 -d":" | fzf --preview="cat {}" --preview-window=right:70%:wrap `
}
esac;;
esac;;
else
echo "a switch is required. -s for search, -n for new"
fi
}
After adding the above I can type "note" followed by a switch, either -n (new) or -s (search) at the command line. With the -n switch I am given a file named with the current date, and date stamped with the same (formatted differently) at the top of the file. With the -s switch followed by search terms in double quotes, my notes are searched for the content within the quotes.
Here are what the lines in the function do:
- cd /home/roger/Documents/notes - change into the directory where my notes are stored. Set this to your desired location.
- if [ $# -gt 0 ]; then - if a switch is present, continue with the process, else, echo a warning
- case $1 in - determine which code to run based on the switch (the first input value)
- -n) if the switch is -n, process the following code
- case "$2" in - handle the second input
- "") - if there is no second switch (it is blank), process the following code
- NAME=$(date +"%F") - set a variable to the current date in the format YYY-MM-DD
- TODAY=$(date +"%A-%b-%d-%Y") - set a variable to the current date in the format Day Name - Month Name - Day of month -year
- touch $NAME - Create a file named with the variable $NAME set above
- echo "$TODAY \n\n" >> $NAME - Write the variable set to $TODAY above and 2 blank lines to the new file
- vim $NAME - Open the file in Vim
- ;; - every case clause must be closed with 2 semicolons
- *) - if the second switch contains anything, process the following code
- NAME=$2 - set a variable = to the second input
- touch $NAME - Create a file named with the variable $NAME set above
- ;; - every case clause must be closed with 2 semicolons
- -s) - We are back to our case for the first switch. If the first input value is -s, process the following code
- case "$2" in - handle the second input
- "") - if there is no second switch (it is blank), process the following code
- vim `fzf --preview="cat {}" --preview-window=right:70%:wrap | sort -k9` - use Vim to open the selected file found using fuzzy find, divide into 2 windows with a preview on the right, sort the results on the 9th column (file creation date)
- ;; - every case clause must be closed with 2 semicolons
- *) - if the second switch contains anything, process the following code
- vim `grep -rnw '/home/roger/Documents/notes' -e $2 | cut -f1 -d":" | fzf --preview="cat {}" --preview-window=right:70%:wrap ` - almost the same as the one above, but grep for the value of the second input
- ;; - every case clause must be closed with 2 semicolons
- the remaining lines close out the cases, handle the else explained above, and close the if
I hope this helps you. I do plan on expanding what I am doing with this functionality.